Application program interface (API) is a set of instructions, protocols, or implementation created to be accessible in a client-side application. It is also used for securely transmitting data when it comes to the database application. It Controls data flow and limits client-side applications to have full control of the main data. A good API can secure the database from being vulnerable to hacking. In this article, we will create a simple Restful API in PHP.
I. Overview
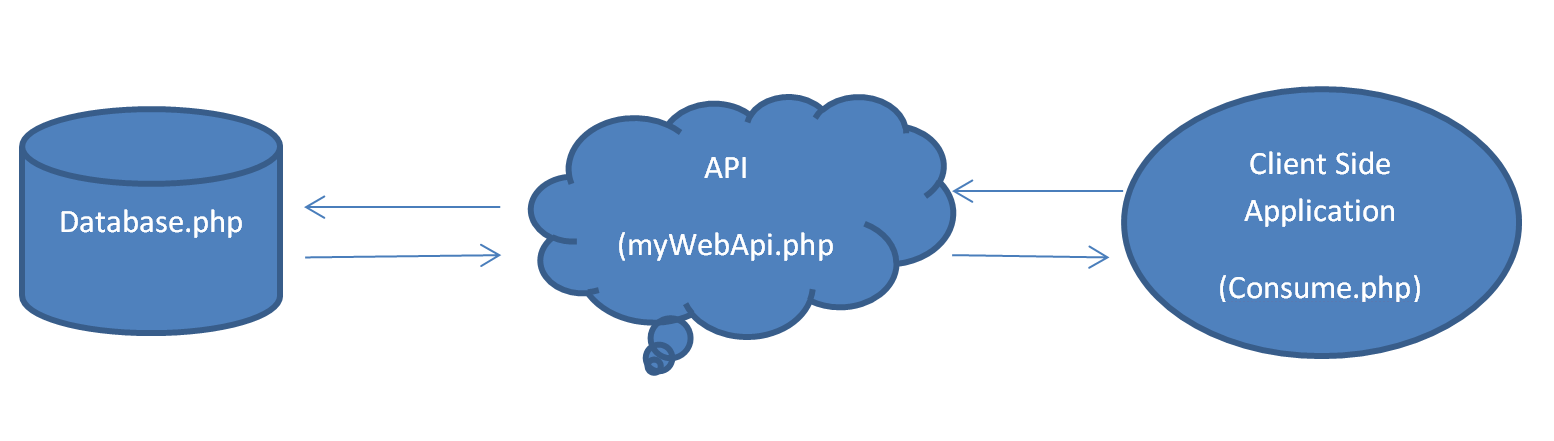
API Concept is to transmit data from a Database and used it in a Client side application like Websites or windows application through our Web API.
Instead of using direct database connection from a server, we are going to use an Array of data to represent our database and return the data using REST API, And get the data using Ajax/JQUERY.
Coding Diagram:
- Database.php » Contain Array of Data to represent our Database
- myWebAPI.php » Represent as Web API
- Consume.php » Represent as our Client side application that consumes our API
In this demo I’ll be using laragon to test the functionality. If you want to try alternative local server for your development you can try follow the article below on how to install apache server on your local machine.
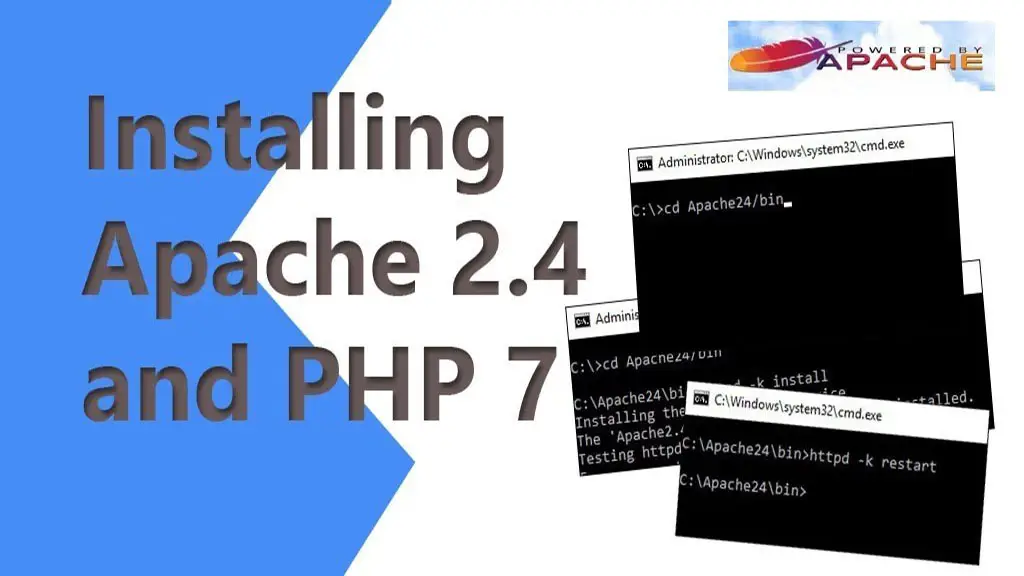
Install Apache Server on Windows machine.
If you want to try Laragon, Download the installer from here..
I. Create Database.php
The code below will ack as our database, but instead of using an actual database we will be using an array of data.
<?php
function get_data ($find){
$data =array("1"=>"Asp.Net",
"2"=>"PHP",
"3"=>"C#");
foreach($data as $data =>$Prog_name)
{
if($data==$find)
{
return $Prog_name;
break;
}
}
}
?>
To explain further, the concept was to get specific value from the array, which in this case, if we pass the value 1 we will get “ASP.Net” value in response.
- $find » Parameter need to be pass to select data from array
Below are the summary of the array we added on our code. This will be the data we will be using to perform a GET operation.
Array Data:
Index | Value |
1 | Asp.Net |
2 | PHP |
3 | C# |
II. Create webapi.php
This file will contain the actual Get API method that we can use to consume from the client side.
<?php
header("Content-Type: application/javascript");
include "Database.php";
if (!empty($_GET["number"])) {
$number = $_GET["number"];
$Lname = get_data($number);
if (empty($Lname)) {
deliver_response(404, "Data not found", null);
} else {
deliver_response(200, "Data found", $Lname);
}
} else {
deliver_response(400, "Invalid request", null);
}
function deliver_response($status, $status_message, $data)
{
$response["status"] = $status;
$response["status_message"] = $status_message;
$response["data"] = $data;
$json_response = json_encode($response);
if (isset($_GET["jsonCallback"])) {
echo $_GET["jsonCallback"] . "(" . $json_response . ")";
} else {
echo $json_response;
}
}
?>
To directly reference the Database.php file it should be place inside the same directory with the webapi.php.
III. Create consume.html
Now, let’s create an html file and use javascript to consume the API method that we created inside webapi.php.
Create another file named webapi.php and use the code below.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Web API Consumer</title>
<script>
function fetchData() {
const number = document.getElementById("number").value;
const jsonCallback = "callbackFunction"; // JSONP callback function name
// Remove existing script tag if any
const existingScript = document.getElementById("jsonpScript");
if (existingScript) {
document.body.removeChild(existingScript);
}
// Create a new script element for JSONP request
const script = document.createElement("script");
script.id = "jsonpScript";
script.src = `webapi.php?number=${number}&jsonCallback=${jsonCallback}`;
// Handle 404 errors gracefully
script.onerror = function() {
const result = document.getElementById("result");
result.innerText = "Error: Data not found (404)";
};
document.body.appendChild(script);
// Define the callback function
window[jsonCallback] = function(response) {
const result = document.getElementById("result");
if (response.status === 200) {
result.innerText = `Data: ${response.data}`;
} else {
result.innerText = `Error: ${response.status_message}`;
}
};
}
</script>
</head>
<body>
<h1>Web API Consumer</h1>
<form onsubmit="event.preventDefault(); fetchData();">
<label for="number">Enter Number:</label>
<input type="text" id="number" name="number" required>
<button type="submit">Fetch Data</button>
</form>
<div id="result"></div>
</body>
</html>
IV. Run Laragon and Test App
Now, to test run Laragon, If you are using a different development server, find the root location and place all your files.
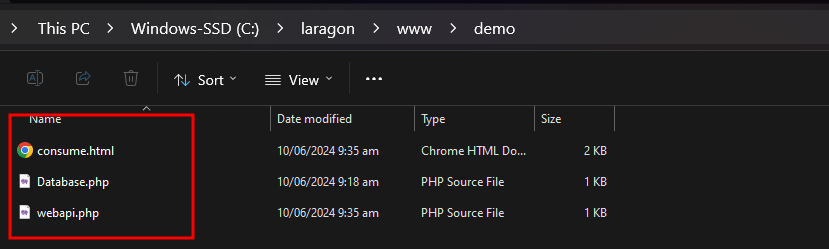
If you are using Laragon, you can directly open the root folder from it’s UI interface.
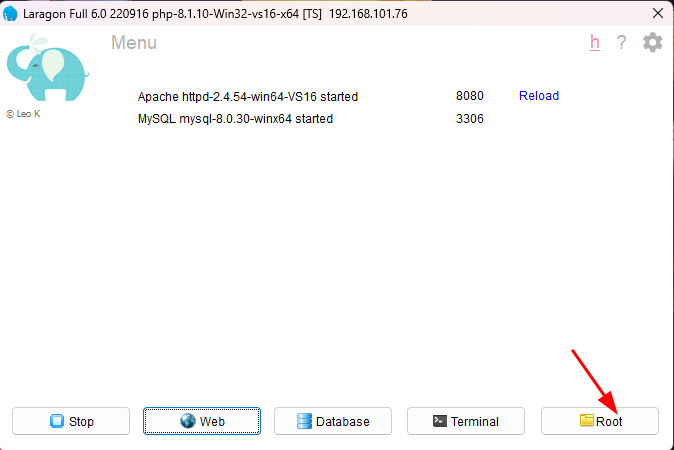
Once you’ve done copying all the necessary file, click Start all and it will start apache server. Then click Web to access your web app in your browser.
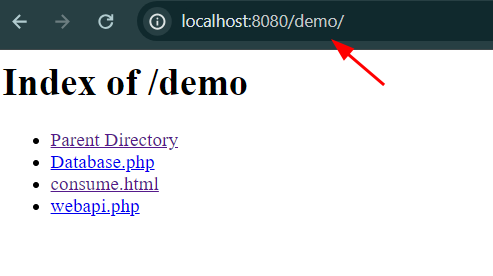
Open consume.html and you can test it.
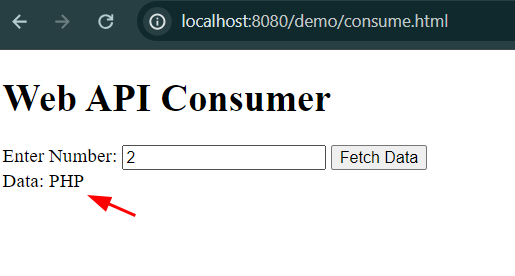
The API method can be access using this URL. It may be using different port on your end but in my case I’m using port 8080.
http://localhost:8080/webapi.php?number=${number}&jsonCallback=${jsonCallback}
Parameters:
- jsonCallback => return the data we request from webapi.php ($_GET[‘jsonCallback’].'(‘.$ json_response.’)’;)
- number => the parameter we pass to the web api method (($_GET[‘number’])))
Source Code
To download our free source code from this tutorial, you can use the button below.
Note: Extract the file using 7Zip and use password: freecodespot
Summary
In this demo, we created a PHP web API from scratch and implemented front-end HTML to consume the REST API. We focused on the GET method for this tutorial, demonstrating the basic steps to create and implement it easily.
I hope this helps you visualize the concept and gives you an idea of how to create a REST API in PHP. Keep Coding!!
You may also try the following article on different ways to create an API in PHP using existing frameworks.
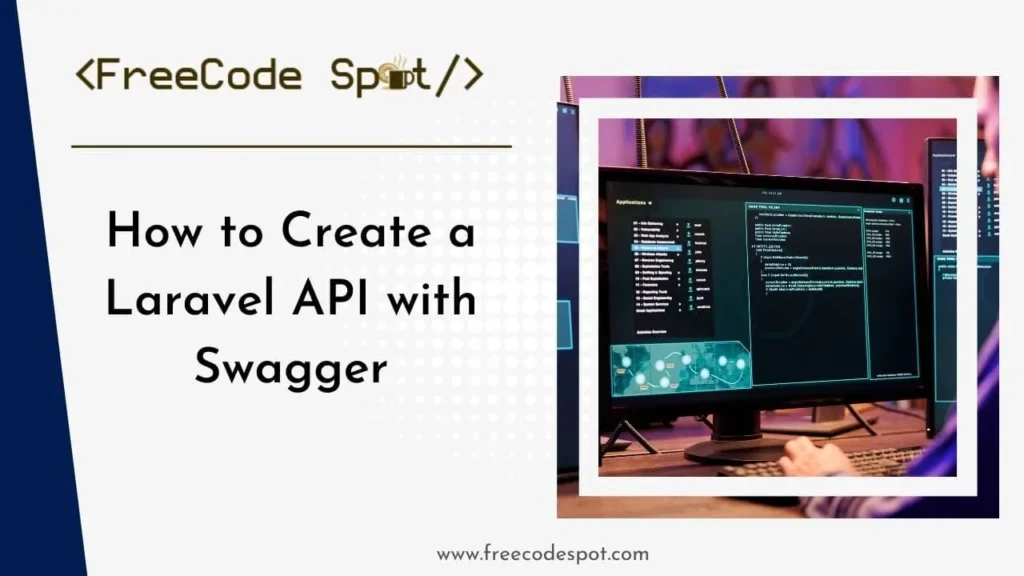
Create API using Laravel Passport
Visit my Blog latest post for more.