What is Angular?
AngularJS is a JavaScript framework that extends HTML attribute to help develop a dynamic Web application.
AngularJS with Asp.Net MVC
Definition of Angular JS from its Official Page:
AngularJS is a structural framework for dynamic web apps. It lets you use HTML as your template language and lets you extend HTML’s syntax to express your application’s components clearly and succinctly. AngularJS’s data binding and dependency injection eliminate much of the code you would otherwise have to write. And it all happens within the browser, making it an ideal partner with any server technology.
To help us understand how this framework works I create a simple asp.net MVC application that uses Angular JS framework to populate HTML table.
Output Preview:
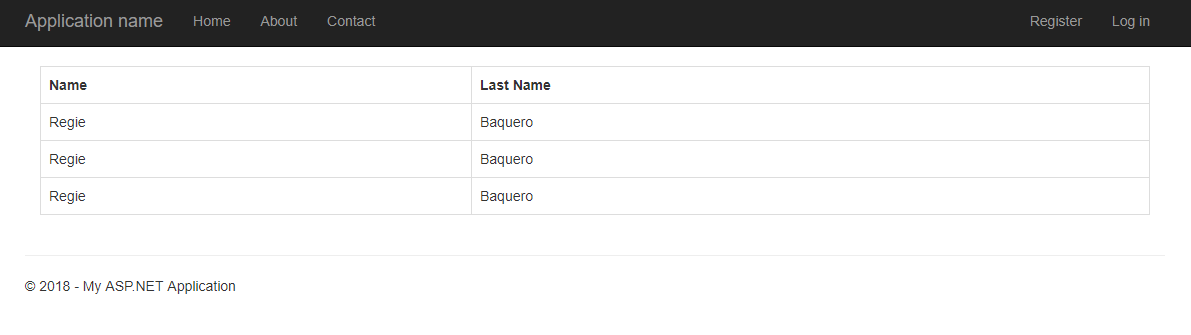
Let’s Start:
Sample Angular JS project with asp.net MVC
First, create a fresh copy of the asp.net MVC Application using Visual Studio. If this is the first time creating one, visit this blog post How To Start with ASP.NET MVC Web Application.
AngularJS with Asp.Net MVC
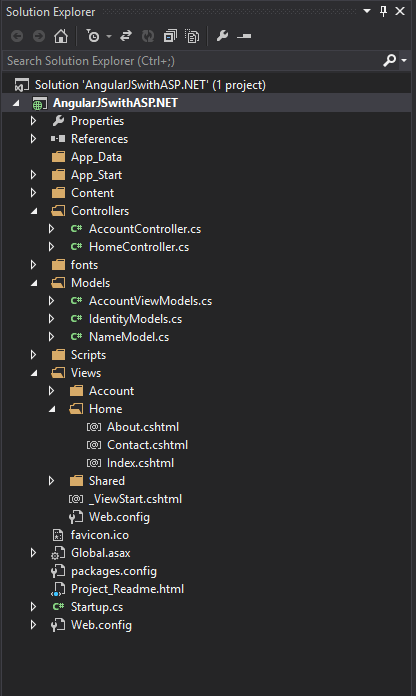
Second, include AngularJS file inside your view (index.cshtml) to use Angular Framework
- <script src=”https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js”></script>
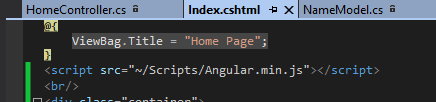
Third, create your HTML table view. If you are using default MVC template of ASP.NET MVC your default controller is HomeController and your default view is located at Home > index.cshtml. Open index file and replace code inside the file using the code below.
Code:
@{
ViewBag.Title = "Home Page";
}
<script src="~/Scripts/Angular.min.js"></script>
<br/>
<div class="container">
<div ng-app="Angular_App" ng-controller="EmpController">
<table class="table table-bordered">
<tr>
<th>Name</th>
<th>Last Name</th>
</tr>
<tr ng-repeat="names in employee">
<td>{{ names.Name }}</td>
<td>{{ names.LastName }}</td>
</tr>
</table>
</div>
</div>
Third, Create new action result in your controller. This will be used for the sample JSON data use to populate to our HTML table. Refer to the code below. I used model binding for my sample JSON data.
Model – NameModel
- Create class inside Model folder this will serve as our model properties for our sample data.
public class NameModel
{
public string Name { get; set; }
public string LastName { get; set; }
}
From your HomeController copy and paste code below.
Sample JSON
[HttpGet]
public ActionResult sample_JSON()
{
List<NameModel> model = new List<NameModel>();
model.Add(new NameModel
{
Name = "FreeCode",
LastName = "Spot"
});
model.Add(new NameModel
{
Name = "FreeCode",
LastName = "Spot"
});
model.Add(new NameModel
{
Name = "FreeCode",
LastName = "Spot"
});
string data = JsonConvert.SerializeObject(model);
return Json(data,JsonRequestBehavior.AllowGet);
}
Fourth, From your view(Index.cshtml) create a script to call this action result (sample_JSON).
Code:
<script>
var app = angular.module('Angular_App', []);
app.controller('EmpController', function ($scope, $http) {
$http({
method: 'GET',
url: '@Url.Action("sample_JSON", "Home")',
headers: {
'Content-type': 'application/json'
}
}).then(function (response) {
$scope.employee = JSON.parse(response.data);
}, function (error) {
console.log(error);
});
});
</script>
For a successful request your call response will look like this:
{"data":"[{\"Name\":\"Regie\",\"LastName\":\"Baquero\"}]","status":200,"config":{"method":"GET","transformRequest":[null],"transformResponse":[null],"jsonpCallbackParam":"callback","url":"/Home/get","headers":{"Accept":"application/json, text/plain, */*"}},"statusText":"OK","xhrStatus":"complete"}
To get the value of the actual data we pass, you can call it using response.data and stringtify( ex. JSON.stringtify(response.data )) to convert into string and output “[{\”Name\”:\”Regie\”,\”LastName\”:\”Baquero\”}]”.
And we’re done. Thank you for reading! Happy Coding!!
You can review your code using the Full Code summary below.
Model:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace AngularJSwithASP.NET.Models
{
public class NameModel
{
public string Name { get; set; }
public string LastName { get; set; }
}
}
Home Controller
using System.Collections.Generic;
using System.Data;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace AngularJSwithASP.NET.Controllers
{
public class HomeController : Controller
{
public ActionResult Index()
{
return View();
}
[HttpGet]
public ActionResult sample_JSON()
{
List<NameModel> model = new List<NameModel>();
model.Add(new NameModel
{
Name = "FreeCode",
LastName = "Spot"
});
model.Add(new NameModel
{
Name = "FreeCode",
LastName = "Spot"
});
model.Add(new NameModel
{
Name = "FreeCode",
LastName = "Spot"
});
string data = JsonConvert.SerializeObject(model);
return Json(data,JsonRequestBehavior.AllowGet);
}
}
}
Index.cshmtl
@{
ViewBag.Title = "Home Page";
}
<script src="~/Scripts/Angular.min.js"></script>
<br/>
<div class="container">
<div ng-app="Angular_App" ng-controller="EmpController">
<table class="table table-bordered">
<tr>
<th>Name</th>
<th>Last Name</th>
</tr>
<tr ng-repeat="names in employee">
<td>{{ names.Name }}</td>
<td>{{ names.LastName }}</td>
</tr>
</table>
</div>
</div>
<script>
var app = angular.module('Angular_App', []);
app.controller('EmpController', function ($scope, $http) {
$http({
method: 'GET',
url: '@Url.Action("sample_JSON", "Home")',
headers: {
'Content-type': 'application/json'
}
}).then(function (response) {
$scope.employee = JSON.parse(response.data);
}, function (error) {
console.log(error);
});
});
</script>