How to submit Forms with validation using AgularJS:
I’m new to AngularJS, and I was trying to create a CRUD application. While I was working on this project, I run into some problem:
“How to submit a form using AngularJS with ASP.NET MVC model binding”
In this article, I would like to share with you a method I found on the internet. To start, you need to create a new MVC Project. If this is your first time creating a project, you can visit this article to guide how to create the ASP.NET Application. Read this link. Create your first ASP.NET Application.
I assume you have already created a project. To have an overview of what view we are about to create, below is my final form view.
Output Preview: – Submit Forms with validation using AgularJS
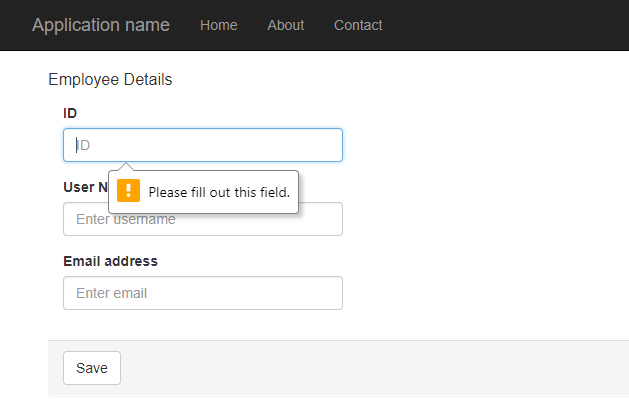
Let’s Start:
First, create a model class. To put every file in place, it is best to put your model class in your Model folder. Then declare all properties you need for your inputs in your forms.
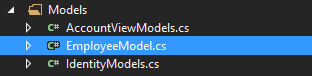
Model Properties:
public class EmployeeModel { public string UserName { get; set; } public string Email { get; set; } public int ID { get; set; } }
Second, create your view. If you are using the default template of ASP.NET MVC, your default controller would probably be HomeController, and your default view is found under View Folder Home > index.chtml. Open the file and replace the code using the snippet below.
- Declare @using AngularCrudOperation.Models > to make model class we created a while ago accessible from the view.
There are two ways of implementing input in the ASP.NET MVC view. You can either use Html Helper or proceed using a plain HTML input.
Inputs > HTML Helper
- @Html.EditorFor(m => m.Email, new { @placeholder = “Enter email”, @class = “form-control”, required = “required”, ng_model = “model.Email” })
Code:
@using AngularCrudOperation.Models @model EmployeeModel @{ ViewBag.Title = "Home Page"; } <br /> <div class="container"> <div ng-app="HomeApp" ng-controller="HomeController"> <form name="employee_form" data-ng-submit="AddEmployee()"> <div class="panel"> <div class="panel-header"> <h4 class="panel-title">Employee Details</h4> </div> <div class="panel-body"> <div class="form-group"> <label for="ID">ID</label> <input type="text" class="form-control" id="ID" placeholder="ID" ng-model="model.ID" required="required"> </div> <div class="form-group"> <label for="username">User Name</label> <input type="text" class="form-control" id="username" placeholder="Enter username" ng-model="model.UserName" required="required"> </div> <div class="form-group"> <label for="Email">Email address</label> <input type="email" class="form-control" id="Email" placeholder="Enter email" ng-model="model.Email" required="required"> </div> </div> <div class="panel-footer"> <button type="submit" class="btn btn-default">Save</button> </div> </div> </form> <br /> </div> </div>
Third, create an AngularJS script for your application. We need to create two functions based on the view we created a while ago.
- model > binding models with AngularJS
- AddEmployee > function used to submit our form input using post request.
Code:
<script src="~/Scripts/Angular.min.js"></script> <script> var app = angular.module('HomeApp', []); app.controller('HomeController', function ($scope, $http) { $scope.model = @Html.Raw(Json.Encode(Model)) $scope.AddEmployee = function () { debugger; var eee = $scope.model; $http({ method: 'POST', url: '@Url.Action("AddEmployee", "Home")', data: $scope.model, headers: { 'Content-type': 'application/json' } }).then(function (response) { $scope.employee = JSON.parse(response.data); }, function (error) { console.log(error); }); } }); </script>
Fourth, create a POST method in your HomeController, where we direct our POST request from our AngularJS App. For the presentation, I created an empty method with no functionality at all. This is to test if we can successfully pass the data into our method AddEmployee. Copy the code below and test if it works.
Code:
[HttpPost] public string AddEmployee(EmployeeModel empdetails) { string result = ""; return result; }
And that is all you need to do to submit a form using angularJS. This is just a part of the CRUD application I was about to create. I was hoping you could stay connected with this blog and check for my latest post to be updated. Thank you for reading happy coding.
Share this post if this helps you!
Code Summary:
Model Class
using System; using System.Collections.Generic; using System.ComponentModel.DataAnnotations; using System.Linq; using System.Web; namespace AngularCrudOperation.Models { public class EmployeeModel { [Required] public string UserName { get; set; } public string Email { get; set; } public int ID { get; set; } } }
HomeController
using AngularCrudOperation.Models; using AngularCrudOperation.Service; using Newtonsoft.Json; using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.Mvc; namespace AngularCrudOperation.Controllers { public class HomeController : Controller { CRUDService service = new CRUDService(); public ActionResult Index() { return View(); } [HttpPost] public string AddEmployee(EmployeeModel empdetails) { string result = "";//service.AddEmployee(empdetails); return result; } } }
Index.cshtml
@using AngularCrudOperation.Models @model EmployeeModel @{ ViewBag.Title = "Home Page"; } <br /> <div class="container"> <div ng-app="HomeApp" ng-controller="HomeController"> <form name="employee_form" data-ng-submit="AddEmployee()"> <div class="panel"> <div class="panel-header"> <h4 class="panel-title">Employee Details</h4> </div> <div class="panel-body"> <div class="form-group"> <label for="ID">ID</label> <input type="text" class="form-control" id="ID" placeholder="ID" ng-model="model.ID" required="required"> </div> <div class="form-group"> <label for="username">User Name</label> <input type="text" class="form-control" id="username" placeholder="Enter username" ng-model="model.UserName" required="required"> </div> <div class="form-group"> <label for="Email">Email address</label> <input type="email" class="form-control" id="Email" placeholder="Enter email" ng-model="model.Email" required="required"> </div> </div> <div class="panel-footer"> <button type="submit" class="btn btn-default">Save</button> </div> </div> </form> <br /> </div> </div> <script src="~/Scripts/Angular.min.js"></script> <script> var app = angular.module('HomeApp', []); app.controller('HomeController', function ($scope, $http) { $scope.model = @Html.Raw(Json.Encode(Model)) $scope.AddEmployee = function () { debugger; var eee = $scope.model; $http({ method: 'POST', url: '@Url.Action("AddEmployee", "Home")', data: $scope.model, headers: { 'Content-type': 'application/json' } }).then(function (response) { $scope.employee = JSON.parse(response.data); }, function (error) { console.log(error); }); } }); </script>