In this comprehensive guide, you’ll Learn ReactJS from Scratch – covering the basics, from understanding React’s advantages to setting up your development environment. Regardless of your level of coding experience, this article will hopefully give you a solid basis for creating cutting-edge online applications using React. Let’s dive into the world of React and start creating!
What is ReactJS?
React, also referred to as ReactJS, is a popular and powerful JavaScript user interface library. React, created and maintained by Facebook, makes it simple for developers to construct dynamic and interactive online apps. Because of its component-based architecture, it is effective for creating reusable user interface elements.
Why should web developers use ReactJS?
- Component-Based Architecture: React organizes UI into components, making it easy to manage and reuse code.
- Virtual DOM: React utilizes a virtual representation of the DOM, significantly improving performance by minimizing direct manipulation of the actual DOM.
- Declarative Syntax: With React, you describe how your UI should look, and React takes care of updating the DOM to match your desired state.
- Community and Ecosystem: React has a vast and active community, providing support, libraries, and tools that enhance the development process.
I. Setting Up Your Development Environment
Before diving into React development, ensure you have Node.js and npm (Node Package Manager) installed on your machine to seamlessly Learn ReactJS from scratch.
1. Visit nodejs.org to download and install the latest version. npm is bundled with Node.js.
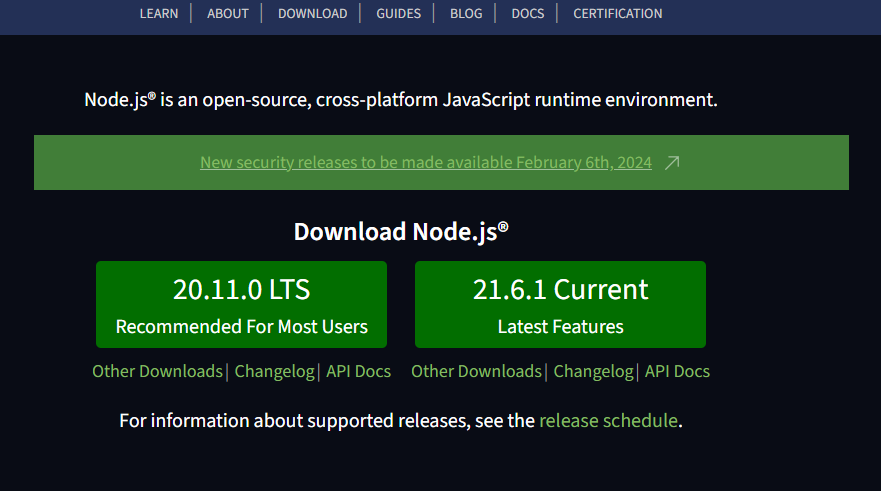
2. Creating a new React project with Create React App
To quickly set up a new React project, use Create React App. Open your terminal and run the following commands:
npx create-react-app my-react-app
cd my-react-app
npm start
This will create a new React application and start a development server. You can access your app at http://localhost:3000.
II. React Folder Structure
When you create a new React app, it generates a specific folder structure. Understanding this structure is crucial for organizing your code effectively. Here are some key folders:
- public: Contains the HTML file and other assets.
- src: Holds your application’s source code.
- components: Store your React components here.
- App.js: The main component that serves as the entry point of your app.
- index.js: Renders the App component into the DOM.
III. Understanding JSX
1. Introduction to JSX syntax
JSX (JavaScript XML) is a syntax extension for JavaScript, used with React to describe what the UI should look like. It looks similar to XML or HTML but allows you to write JavaScript expressions within the code. JSX makes it easier to create and visualize components in a more readable manner.
2. JSX vs. HTML
While JSX resembles HTML, there are differences. JSX allows embedding dynamic expressions within curly braces {}, facilitating the integration of JavaScript logic directly into the markup. It gets compiled to JavaScript and is more powerful than traditional HTML, especially when dealing with dynamic content and React components.
// JSX
import React from 'react';
const JSXExample = () => {
const dynamicContent = 'Hello, React!';
return (
<div>
<h1>{dynamicContent}</h1>
<p>This is a JSX example.</p>
</div>
);
};
export default JSXExample;
In the JSX example above, you can see how JavaScript expressions, in this case, the variable dynamicContent, can be embedded within curly braces {}. This allows dynamic content to be easily integrated into the JSX code.
Now, let’s contrast this with the equivalent HTML code:
<!-- HTML Equivalent -->
<div>
<h1>Hello, React!</h1>
<p>This is an HTML equivalent example.</p>
</div>
In the HTML example, you lack the ability to embed dynamic JavaScript expressions directly. JSX provides a more expressive and dynamic way of building components, making it a powerful tool for React developers.
Summary
In conclusion, ReactJS is a fantastic library for building modern, efficient, and maintainable web applications. With the right foundation, including a solid development environment setup, understanding folder structures, and mastering JSX syntax, you’re well on your way to becoming a proficient React developer.
Keep coding!
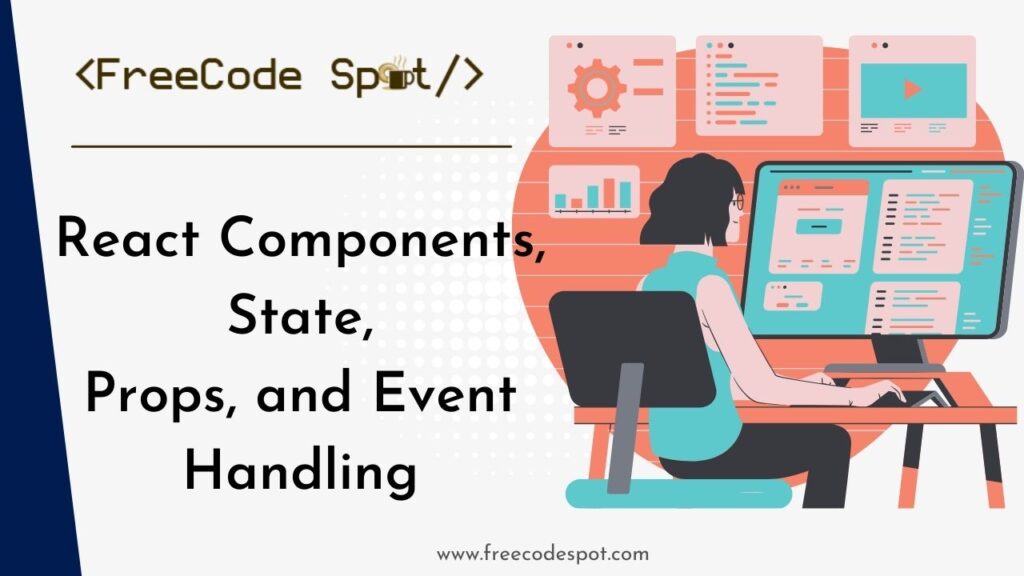
Next, check this out!! React Component, State, Props, and Event Handling
Visit the Blog Page for more programming posts.