The WebClient class in C# is a part of the .NET framework and is commonly used for making web requests to interact with remote servers. It provides a simple way to download or upload data, send GET and POST requests, and handle web responses. This article will explore the syntax of WebClient requests in C#, showcasing basic and advanced usage, best practices, common issues, and troubleshooting tips.
Basic Syntax and Examples
Creating and Using a WebClient Object
To start using WebClient, you need to create an instance of the WebClient class. Here’s the basic syntax:
using System.Net;
WebClient webClient = new WebClient();
Downloading Data
- Downloading a String
You can download data from a URL as a string using the DownloadString method:
string url = "https://example.com/data";
string data = webClient.DownloadString(url);
Console.WriteLine(data);
- Downloading a File
To download a file from a URL and save it locally, use the DownloadFile method:
string url = "https://example.com/file.zip";
string filePath = @"C:\path\to\save\file.zip";
webClient.DownloadFile(url, filePath);
Uploading Data
- Uploading a String
You can upload data to a server as a string using the UploadString method:
string url = "https://example.com/upload";
string dataToSend = "data to upload";
string response = webClient.UploadString(url, dataToSend);
Console.WriteLine(response);
- Uploading a File
To upload a file to a server, use the UploadFile method:
string url = "https://example.com/upload";
string filePath = @"C:\path\to\file.zip";
byte[] response = webClient.UploadFile(url, filePath);
Console.WriteLine(System.Text.Encoding.UTF8.GetString(response));
Sending WebClient Http Requests
- GET Request
Sending a GET request to retrieve data from a server
string url = "http://api/service";
using (var client = new WebClient())
{
client.Headers.Add("content-type", "application/json");
string response = client.DownloadString(url);
}
- POST Request
Uploads the specified string to the specified resource, using the POST method.
public string UploadString (string address, string? method, string data);
Sample Code:
string param = "{"data1":"value1","data2":"value2"}";
string url = "http://api/service";
using (var client = new WebClient())
{
client.Headers.Add("content-type", "application/json");
string response = Encoding.ASCII.GetString(client.UploadData(url, "POST", Encoding.UTF8.GetBytes(param)));
}
- DELETE Request
DELETE request allows for the removal of resources on a server
Notes:
- URL » Replace
"http://api/service"
with the actual URL of the resource you want to delete. - Headers » Adjust headers (
Content-Type
or others) as per your API’s requirements. - Error Handling » Implement robust error handling to manage various scenarios effectively.
string url = "http://api/service";
using (var client = new WebClient())
{
client.Headers.Add("Content-Type", "application/json");
// Send the DELETE request
byte[] response = client.UploadData(url, "DELETE", new byte[0]);
// Convert the response to a string (if needed) and display it
string result = Encoding.ASCII.GetString(response);
Console.WriteLine("Response: " + result);
}
- PUT Request
PUT request enables the updating or creation of resources on a server
string param = "{"data1":"value1","data2":"value2"}";
string url = "http://api/service";
using (var client = new WebClient())
{
client.Headers.Add("content-type", "application/json");
string response = client.UploadString(url, "PUT", param);
}
Advanced Usage
Setting Headers
You can add custom headers to your requests using the Headers property:
webClient.Headers.Add("User-Agent", "Mozilla/5.0 (Windows NT 10.0; Win64; x64)");
webClient.Headers.Add("Content-Type", "application/x-www-form-urlencoded");
Handling Responses
To handle responses, especially when dealing with byte arrays, you can use methods like DownloadData.
byte[] data = webClient.DownloadData("https://example.com/data");
Console.WriteLine(System.Text.Encoding.UTF8.GetString(data));
Managing Asynchronous Requests
For non-blocking operations, use asynchronous methods such as DownloadStringAsync
and UploadStringAsync
.
webClient.DownloadStringCompleted += (sender, e) =>
{
Console.WriteLine(e.Result);
};
webClient.DownloadStringAsync(new Uri("https://example.com/data"));
Best Practices
Error Handling
Implement proper error handling using try-catch blocks to manage exceptions like WebException
.
try
{
string response = webClient.DownloadString("https://example.com/data");
Console.WriteLine(response);
}
catch (WebException ex)
{
Console.WriteLine($"Error: {ex.Message}");
}
Security Considerations
- Use HTTPS: Ensure all requests use HTTPS to encrypt data.
- Validate Input: Sanitize and validate any data being sent or received.
Dispose WebClient: Always dispose of the WebClient instance after use to free resources.
using (WebClient webClient = new WebClient())
{
// Use webClient
}
Performance Tips
- Reuse WebClient: For multiple requests, reuse the WebClient instance instead of creating new ones.
- Set Timeouts: Although WebClient does not support direct timeout settings, consider using HttpClient for more control over timeouts and retries.
Comparison with HttpClient and WebRequest
- WebClient: Easy to use for simple requests but limited in functionality.
- HttpClient: More flexible and robust, supports async/await, better error handling, and customization.
- WebRequest: More control over the request but more complex and verbose.
Common Issues and Troubleshooting
Timeout Issues
If a request takes too long, consider switching to HttpClient for better timeout management.
SSL/TLS Errors
Ensure the server supports modern SSL/TLS protocols and consider updating the .NET framework version if necessary.
Handling Large Data
For large data transfers, consider using HttpClient with streaming to manage memory usage more efficiently.
Authentication Issues
Ensure proper authentication headers are set and validate credentials.
webClient.Headers[HttpRequestHeader.Authorization] = "Bearer your_token_here";
Summary
The WebClient class in C# provides a straightforward way to make web requests, download and upload data, and handle responses. While it’s suitable for simple tasks, for more advanced scenarios, HttpClient is often the better choice due to its flexibility and performance. By following best practices and understanding common issues, you can effectively use WebClient in your C# applications.
For further reading, refer to the official Microsoft documentation on WebClient.
Keep Coding!!
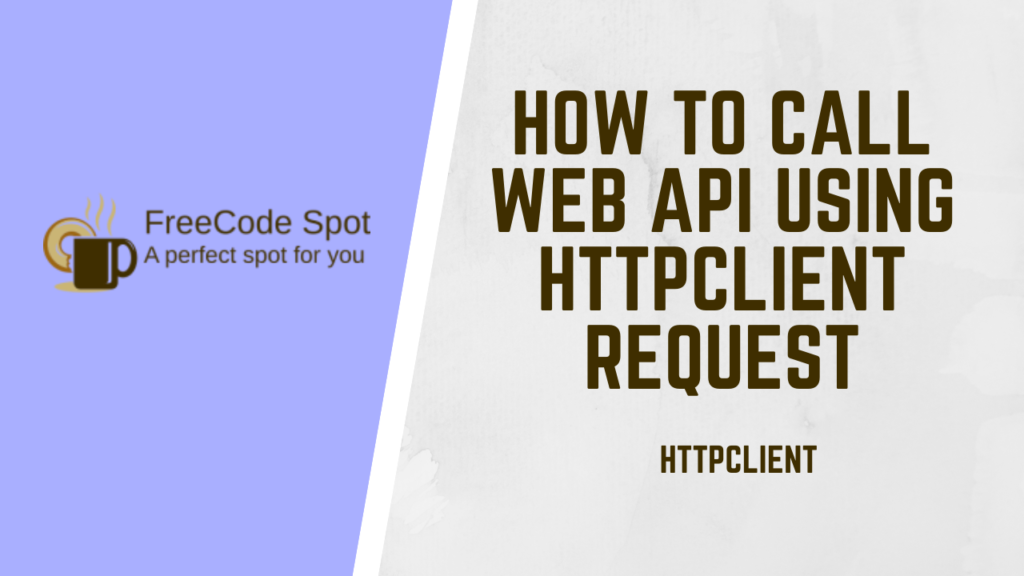
Make Http Request using HttpClient. » Read More.