In this tutorial, we are going to create a sample AngularJS Application with ASP NET MVC. We will create a new project that will implement a full CRUD operation. The scenario would be to use ASP.NET MVC as backend concept and use Angular with the client-side interaction. This article is designed for beginners to see how we can easily integrate AngularJS with ASP.NET MVC.
See Also : Angular 11 Tutorial
What is AngularJS?
AngularJS is a front end JavaScript MVC framework that is to develop dynamic web applications. This framework lets you use HTML as your template language and enables you to extend HTML’s syntax to express your application’s components clearly. AngularJS’s data binding and dependency injection eliminate much of the code you are used to writing.
Before we start please make sure that you have all the tools needed for this tutorial.
- The latest version of Visual Studio
- SQL Server
If all of the tools listed above are already installed on your machine, let’s proceed with the steps below.
I. Create a new ASP.NET Web Application
- First, create your ASP.NET Web Application. To do that just follow the steps below.
- Select File > New > Project.
- Select ASP.NET Web Application(.NET Framework). Name the project AngularJS_Demo to have the same namespace as my project. Then select the latest .NET Framework version, which in my case it’s 4.7.2. Click OK.
- Select an MVC template and then uncheck Configure for HTTPS.
- Lastly, Click on Create.
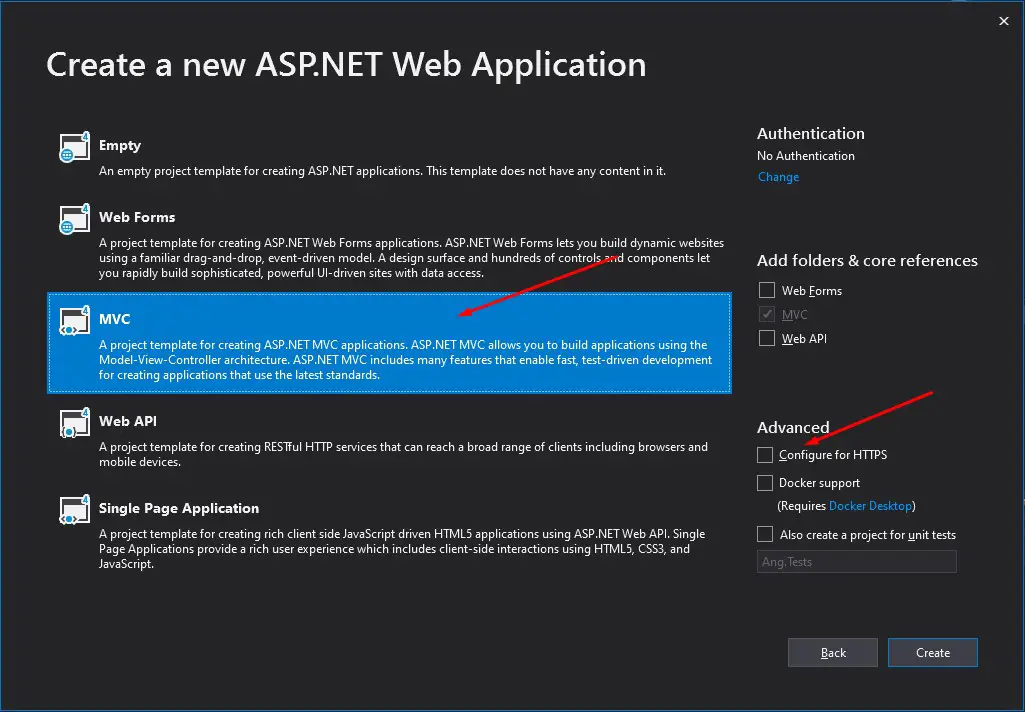
2. Wait for Visual studio to generate need files for your project. Once done, your project solution will look like this.
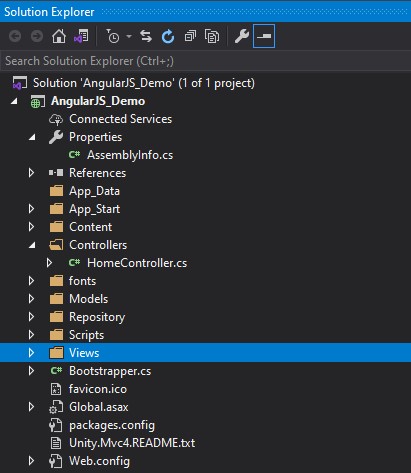
II. Setup SQL Database
Now that we have our new Web Application created let’s make our database table and SQL Stored Procedure for these projects. You can use the below for your reference.
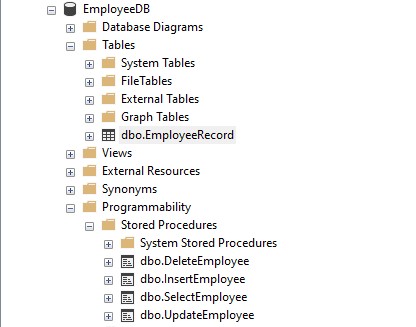
- Create your SQL Database and create a table containing the columns from the script below. Or copy the SQL query below.
USE [EmployeeDB]
GO
SET ANSI_NULLS ON
GO
SET QUOTED_IDENTIFIER ON
GO
CREATE TABLE [dbo].[EmployeeRecord](
[ID] [int] IDENTITY(1,1) NOT NULL,
[Name] nvarchar NULL,
[Email] nvarchar NULL
) ON [PRIMARY]
GO
2. Now, let’s create the SQL Stored procedure CRUD operation. You may copy and execute the query list below.
[dbo].[InsertEmployee]
Create Employee record
USE [EmployeeDB]
GO
SET ANSI_NULLS ON
GO
SET QUOTED_IDENTIFIER ON
GO
CREATE PROCEDURE [dbo].[InsertEmployee]
@Name nvarchar(50),
@Email nvarchar(50)
AS
BEGIN
SET NOCOUNT ON;
INSERT INTO EmployeeRecord VALUES(@Name, @Email)
END
[dbo].[SelectEmployee]
Read Employee Records
USE [EmployeeDB]
GO
SET ANSI_NULLS ON
GO
SET QUOTED_IDENTIFIER ON
GO
Create PROCEDURE [dbo].[SelectEmployee]
AS
BEGIN
SET NOCOUNT ON; Select * FROm EmployeeRecord
END
[dbo].[UpdateEmployee]
Update Employee Record
USE [EmployeeDB]
GO
SET ANSI_NULLS ON
GO
SET QUOTED_IDENTIFIER ON
GO
CREATE PROCEDURE [dbo].[UpdateEmployee]
@ID int ,
@Name nvarchar(50),
@Email nvarchar(50)
AS
BEGIN
SET NOCOUNT ON;
Update EmployeeRecord set Name = @Name , Email = @Email WHERE ID = @ID
END
[dbo].[DeleteEmployee]
Delete Employee Record
USE [EmployeeDB]
GO
SET ANSI_NULLS ON
GO
SET QUOTED_IDENTIFIER ON
GO
CREATE PROCEDURE [dbo].[DeleteEmployee]
@ID int
AS
BEGIN
SET NOCOUNT ON;
Delete From EmployeeRecord WHERE ID = @ID
END
III. Create a Repository Class
This class will the handle the call to our SQL Stored Procedure. This will serve as our services method for our AngularJS Framework.
- Before we start creating our repository, create a model property class. You can place it inside the Models folder. See the code snippet below.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace AngularJS_Demo.Models
{
public class EmployeeModel
{
public int ID { get; set; }
public string Name { get; set; }
public string Email { get; set; }
}
}
2. Add your database connection string on your Web.config. To do that, open web.config and add Connectionstring tags. See the code snippet below.
To access connection string from any class you can use the code below.
string connectionString = ConfigurationManager.ConnectionStrings["myConnectionString"].ConnectionString;
Web.config <Connectionstrings> tags:
<connectionStrings>
<add name="myConnectionString" connectionString="server=DESKTOP-4DU98BI\SQLEXPRESS01;database=EmployeeDB;uid=freecode;password=freecodespot;" />
</connectionStrings>
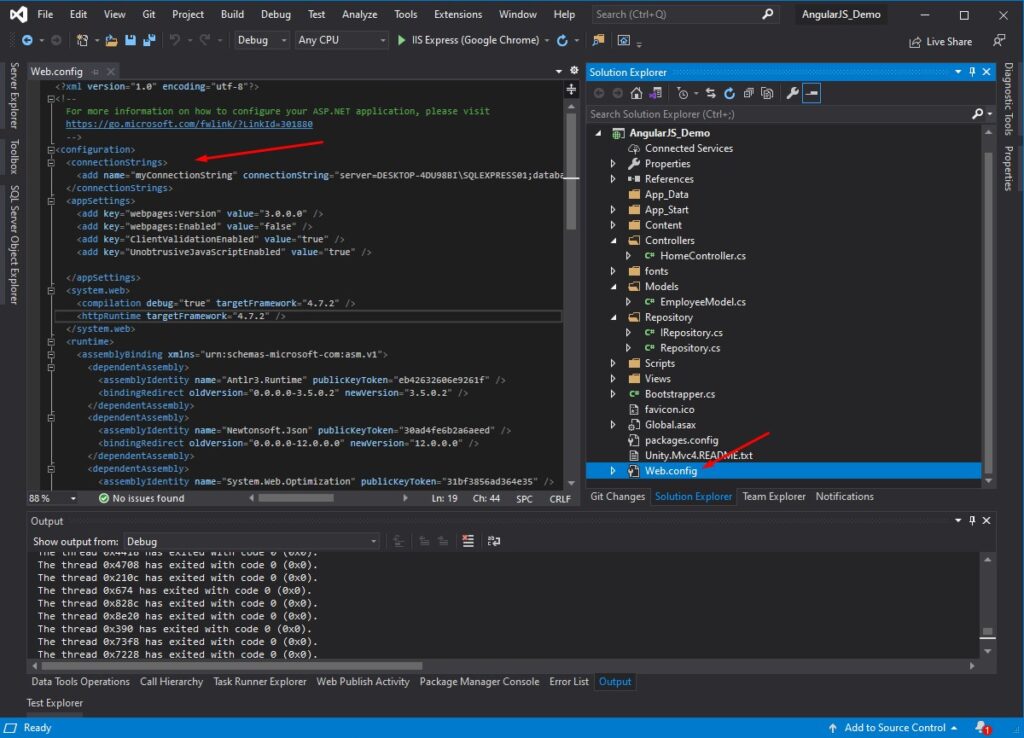
3. Now, Create a Repository folder on your project solution root directory. Create two files, an Interface and a C# class. Please refer to the image shown below.
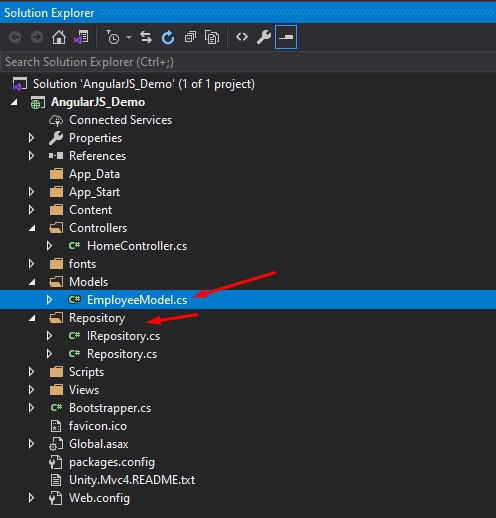
- IRepository » Interface class
- Repository » Implementation of the interface class
4. Open IRepository class, we will add the necessary interface method for our CRUD operation. Copy the code snippet below.
using AngularJS_Demo.Models;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace AngularJS_Demo.Repository
{
public interface IRepository
{
List GetEmployees();
string AddEmployee(EmployeeModel empDetails);
string updateEmployee(EmployeeModel empdetails);
string deleteEmployee(int id); }
}
5. In the Repository class copy the CRUD implementation below. This code contains four method.
- AddEmployee » [dbo].[InsertEmployee]
- GetEmployees » [dbo].[SelectEmployee]
- updateEmployee » [dbo].[UpdateEmployee]
- deleteEmployee » [dbo].[DeleteEmployee]
using AngularJS_Demo.Models;
using System;
using System.Collections.Generic;
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
using System.Linq;
using System.Web;
namespace AngularJS_Demo.Repository
{
public class Repository : IRepository
{
private string connectionString = ConfigurationManager.ConnectionStrings["myConnectionString"].ConnectionString; // "Data Source=LAPTOP-JD2CG4N8;Initial Catalog=EmployeeDB;Integrated Security=True;Connect Timeout=15;Encrypt=False;TrustServerCertificate=False";
public string AddEmployee(EmployeeModel empDetails)
{
string response = "";
using (SqlConnection conn = new SqlConnection(connectionString))
{
SqlCommand cmd = new SqlCommand("InsertEmployee", conn);
cmd.Parameters.Add("@Name", SqlDbType.NVarChar, 50).Value = empDetails.Name;
cmd.Parameters.Add("@Email", SqlDbType.NVarChar, 50).Value = empDetails.Email;
cmd.CommandType = CommandType.StoredProcedure;
conn.Open();
cmd.ExecuteNonQuery();
response = "Success";
conn.Close();
}
return response;
}
public string deleteEmployee(int id)
{
string response = "";
using (SqlConnection conn = new SqlConnection(connectionString))
{
SqlCommand cmd = new SqlCommand("DeleteEmployee", conn);
cmd.Parameters.Add("@ID", SqlDbType.Int).Value = id;
cmd.CommandType = CommandType.StoredProcedure;
conn.Open();
cmd.ExecuteNonQuery();
response = "Success";
conn.Close();
}
return response;
}
public List<EmployeeModel> GetEmployees()
{
List<EmployeeModel> list = new List<EmployeeModel>();
using (SqlConnection conn = new SqlConnection(connectionString))
{
SqlCommand cmd = new SqlCommand("dbo.SelectEmployee", conn);
cmd.CommandType = System.Data.CommandType.StoredProcedure;
SqlDataAdapter da = new SqlDataAdapter(cmd);
DataSet ds = new DataSet();
da.Fill(ds);
if (ds.Tables.Count > 0)
{
if (ds.Tables[0].Rows.Count > 0)
{
foreach (DataRow row in ds.Tables[0].Rows)
{
list.Add(new EmployeeModel
{
Name = row["Name"].ToString().Trim(),
Email = row["Email"].ToString().Trim(),
ID = int.Parse(row["ID"].ToString())
});
}
}
}
}
return list;
}
public string updateEmployee(EmployeeModel empdetails)
{
string response = "";
using (SqlConnection conn = new SqlConnection(connectionString))
{
SqlCommand cmd = new SqlCommand("UpdateEmployee", conn);
cmd.Parameters.Add("@ID", SqlDbType.Int).Value = empdetails.ID;
cmd.Parameters.Add("@Name", SqlDbType.NVarChar, 50).Value = empdetails.Name;
cmd.Parameters.Add("@Email", SqlDbType.NVarChar, 50).Value = empdetails.Email;
cmd.CommandType = CommandType.StoredProcedure;
conn.Open();
cmd.ExecuteNonQuery();
response = "Success";
conn.Close();
}
return response;
}
}
}
IV. Configure Dependency Injection for ASP.NET MVC
Since I used Dependency Injection on this project, I need to add a few configurations. Just follow the few steps below.
- Add Unity.Mvc4 from your NuGet Package Manager.
Unity is a lightweight, extensible dependency injection container with optional support for instance and type interception.
2. Installation of this package will include a static files name bootstrapper.cs. We need to initialize this inside Global.asax found on the root directory of our project. To initialize add the snippet below.
Bootstrapper.Initialise();
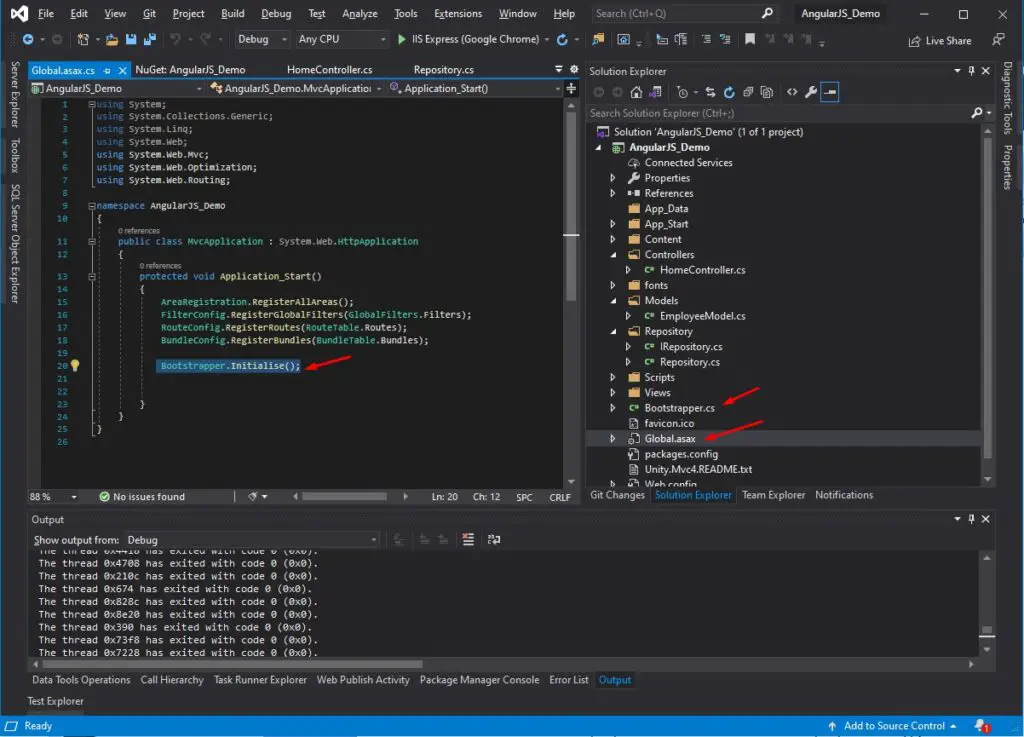
3. Now, open Bootstrapper.cs and register a container by declaring the code snippet below under BuildUnityContainer() method. This code will map our IRepository and Repository class.
container.RegisterType<IRepository, Repository.Repository>();
Bootstrapper class full code:
using System.Web.Mvc;
using AngularJS_Demo.Repository;
using Microsoft.Practices.Unity;
using Unity.Mvc4;
namespace AngularJS_Demo
{
public static class Bootstrapper
{
public static IUnityContainer Initialise()
{
var container = BuildUnityContainer();
DependencyResolver.SetResolver(new UnityDependencyResolver(container));
return container;
}
private static IUnityContainer BuildUnityContainer()
{
var container = new UnityContainer();
// register all your components with the container here
// it is NOT necessary to register your controllers
container.RegisterType<IRepository, Repository.Repository>();
// e.g. container.RegisterType<ITestService, TestService>();
RegisterTypes(container);
return container;
}
public static void RegisterTypes(IUnityContainer container)
{
}
}
}
V. Setup HomeController method for AngularJS
This time let’s create a method from our HomeController for AngularJS consumption. To do that just copy the code snippet below.
First, we need to create a constructor and inject the IRepository class. If you remember, this class handles the call to our SQL Stored Procedure. This is how you can declare it.
private readonly IRepository _repository;
public HomeController(IRepository repository)
{
_repository = repository;
}
Now, add the code snippet below. This method consumes the Repository method that we created awhile ago.
[HttpGet]
public ActionResult getEmployee()
{
List<EmployeeModel> list = new List<EmployeeModel>();
list = _repository.GetEmployees();
string json_data = JsonConvert.SerializeObject(list);
return Json(json_data, JsonRequestBehavior.AllowGet);
}
[HttpPost]
public ActionResult AddEmployee(EmployeeModel empdetails)
{
string result = _repository.AddEmployee(empdetails);
List<EmployeeModel> list = new List<EmployeeModel>();
list = _repository.GetEmployees();
string json_data = JsonConvert.SerializeObject(list);
return Json(json_data, JsonRequestBehavior.AllowGet);
}
[HttpPost]
public ActionResult UpdateEmployee(EmployeeModel empdetails)
{
string result = _repository.updateEmployee(empdetails);
List<EmployeeModel> list = new List<EmployeeModel>();
list = _repository.GetEmployees();
string json_data = JsonConvert.SerializeObject(list);
return Json(json_data, JsonRequestBehavior.AllowGet);
}
[HttpPost]
public ActionResult DeleteEmployee(int ID)
{
string result = _repository.deleteEmployee(ID);
List<EmployeeModel> list = new List<EmployeeModel>();
list = _repository.GetEmployees();
string json_data = JsonConvert.SerializeObject(list);
return Json(json_data, JsonRequestBehavior.AllowGet);
}
VI. Set up AngularJS
To configure AngularJS navigate to your View, which in my case it is the index inside my HomeController.
Note: To use AngularJS you can download the JS file from there official website or use the link below.
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script>
- From your HomeController right-click on index method then choose Go to View.
- Once, you are in the index.cshtml. Copy the code below.
This is the html view that is extended to AngularJS functionality.
@model AngularJS_Demo.Models.EmployeeModel
@{
ViewBag.Title = "Home Page";
}
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script>
<div ng-app="HomeApp" ng-controller="HomeController">
<hr />
<div class="header-info">
<label class="">Employee Details</label>
<button class="btn btn-primary pull-right" data-toggle="modal" data-target="#AddModal" ng-click="clearModel()">Add Employee</button>
</div>
<hr />
<br />
<div class="alert alert-success" ng-if="message">
<a href="#" class="close" data-dismiss="alert" aria-label="close">×</a>
{{message}}
</div>
<div class="table-data">
<table class="table table-hover">
<thead>
<tr>
<th>ID</th>
<th>Full Name</th>
<th>Email Address</th>
<th>Edit</th>
<th>Delete</th>
</tr>
</thead>
<tbody>
<tr ng-repeat="names in employee">
<td>{{ names.ID }}</td>
<td>{{ names.Name }}</td>
<td>{{ names.Email }}</td>
<td><button class="btn btn-info" data-toggle="modal" data-target="#EditModal" ng-click="selectUser(names)">Edit</button></td>
<td><button class="btn btn-danger" ng-click="DeleteEmployee(names)">Delete</button></td>
</tr>
</tbody>
</table>
</div>
<!-- Modal Add modal-->
<div class="modal fade" id="AddModal" role="dialog">
<div class="modal-dialog">
<!-- Modal content-->
<div class="modal-content">
<form name="employee_form" data-ng-submit="AddEmployee()">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal">×</button>
<h4 class="modal-title">Add Employee Details</h4>
</div>
<div class="modal-body">
<div class="form-group">
<label for="exampleInputEmail1">User Name</label>
<input type="text" class="form-control" id="fullname" aria-describedby="emailHelp" placeholder="Enter Full Name" ng-model="model.Name" required="required">
</div>
<div class="form-group">
<label for="exampleInputEmail1">Email address</label>
<input type="email" class="form-control" id="exampleInputEmail1" aria-describedby="emailHelp" placeholder="Enter email" ng-model="model.Email" required="required">
<small id="emailHelp" class="form-text text-muted">We'll never share your email with anyone else.</small>
</div>
</div>
<div class="modal-footer">
<button type="submit" class="btn btn-default pull-left">Save</button>
<button type="button" class="btn btn-default" data-dismiss="modal">Close</button>
</div>
</form>
</div>
</div>
</div>
<!-- Edit modal-->
<div class="modal fade" id="EditModal" role="dialog">
<div class="modal-dialog">
<!-- Modal content-->
<div class="modal-content">
<form name="employee_form" data-ng-submit="UpdateEmployee()">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal">×</button>
<h4 class="modal-title">Edit Employee Details</h4>
</div>
<div class="modal-body">
<div class="form-group">
<label for="exampleInputEmail1">User Name</label>
<input type="text" class="form-control" id="fullname" aria-describedby="emailHelp" placeholder="Enter FullName" ng-model="selectedUser.Name" required="required">
</div>
<div class="form-group">
<label for="exampleInputEmail1">Email address</label>
<input type="email" class="form-control" id="exampleInputEmail1" aria-describedby="emailHelp" placeholder="Enter email" ng-model="selectedUser.Email" required="required">
<small id="emailHelp" class="form-text text-muted">We'll never share your email with anyone else.</small>
</div>
</div>
<div class="modal-footer">
<button type="submit" class="btn btn-primary pull-left">Save</button>
<button type="button" class="btn btn-danger" data-dismiss="modal">Close</button>
</div>
</form>
</div>
</div>
</div>
</div>
3. Now, last step would be to create the script for the AngularJS action. Please see the code below.
<script>
var app = angular.module('HomeApp', []);
app.controller('HomeController', function ($scope, $http) {
$scope.model = @Html.Raw(Json.Encode(Model))
$http({
method: 'GET',
url: '@Url.Action("getEmployee", "Home")',
headers: {
'Content-type': 'application/json'
}
}).then(function (response) {
debugger;
$scope.employee = JSON.parse(response.data);
}, function (error) {
console.log(error);
});
$scope.AddEmployee = function () {
debugger;
var eee = $scope.model;
$http({
method: 'POST',
url: '@Url.Action("AddEmployee", "Home")',
data: $scope.model,
headers: {
'Content-type': 'application/json'
}
}).then(function (response) {
$scope.employee = JSON.parse(response.data);
$scope.message = "Employee added Successfully";
$("#AddModal").modal("hide");
}, function (error) {
console.log(error);
});
}
$scope.selectUser = function (names) {
$scope.selectedUser = names;
}
$scope.UpdateEmployee = function () {
var eee = $scope.selectedUser;
$http({
method: 'POST',
url: '@Url.Action("UpdateEmployee", "Home")',
data: $scope.selectedUser,
headers: {
'Content-type': 'application/json'
}
}).then(function (response) {
$scope.employee = JSON.parse(response.data);
$scope.message = "Employee updated Successfully";
$("#EditModal").modal("hide");
}, function (error) {
console.log(error);
});
}
$scope.DeleteEmployee = function (names) {
$http({
method: 'POST',
url: '@Url.Action("DeleteEmployee", "Home")',
data: names,
headers: {
'Content-type': 'application/json'
}
}).then(function (response) {
$scope.employee = JSON.parse(response.data);
$scope.message = "Employee Deleted Successfully";
$("#EditModal").modal("hide");
}, function (error) {
console.log(error);
});
}
$scope.clearModel = function () {
$scope.model = null;
}
});
</script>
At this point, we can try to run our application. If you encounter some error, I will attach my source code for this tutorial here at my GitHub Account coderbugzz.
The video clip below is the final output:
Summary
This tutorial shows you how you can create a sample AngularJS Application with ASP NET MVC. We also make a CRUD Operation using a SQL Stored Procedure. We also use a Repository pattern and use a third-party extension Unity.MVC3 to configure dependency injection on ASP.NET MVC. We also use native SQL Command to call SQL Stored procedure. Lastly, we use AngularJS on our front-end. I hope you get some ideas here and hopefully help you with your ongoing or future projects.
KEEP Coding!!