This article will show you how to Create and Render Partial Views in ASP NET MVC. One of the best ways to implement a dynamic table without loading the whole page is using the Jquery in Ajax request. Asp.Net MVC offers Ajax helpers to achieve this functionality quickly. Partial View in Asp.Net MVC is one of its strengths. In this tutorial, we are going to use Ajax.Actionlink() to load Partial View in MVC.
Before we start, Please make sure to have installed the following
- The latest version of Visual Studio – You can download it here.
Let’s start:
Funal output:
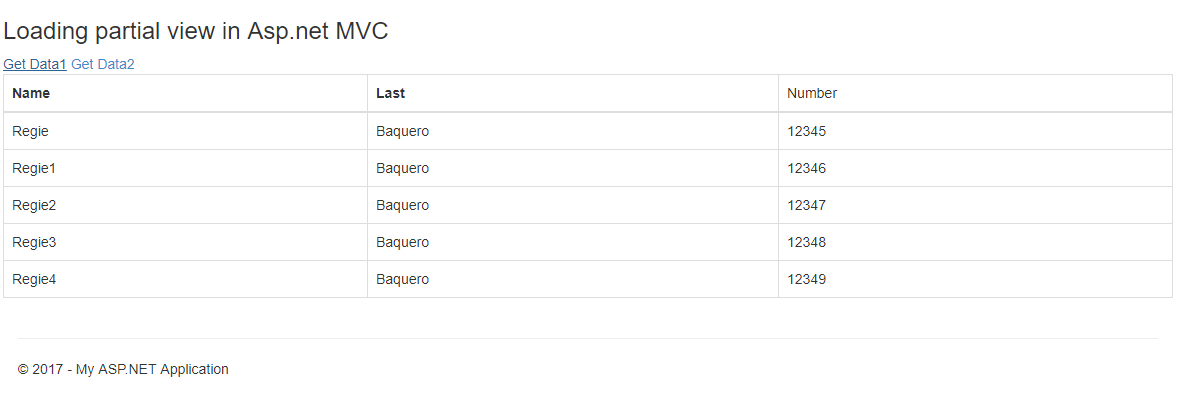
If this is your first time creating an MVC Web Application project, refer to the thread below
• How to start with ASP.NET MVC Web Application
• ASP.NET MVC File structure
First, create a new model class for our model binding in creating a list of our sample data later in this tutorial. In my case, I name it as myModels.cs.
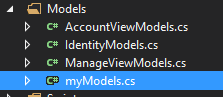
Declare following properties in your model class
public string Name { get; set; }
public string LastName { get; set; }
public string Number { get; set; }
Create a partial view inside View->Shared folder
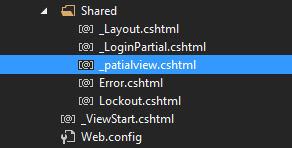
• To add, right-click on the Shared folder under Views and select Add->New Item, and a new window will pop-up. Select Web from the window’s left pane, then choose MVC 5 View Page(Razor) in the middle pane. Name your view. In my case, I name it as _partialview.
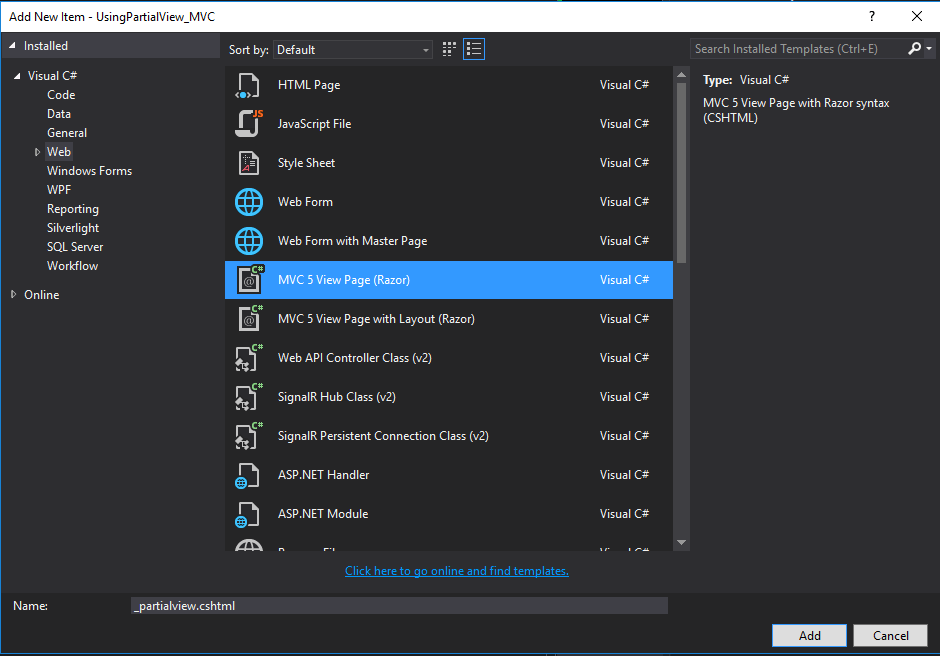
This partial view will contain table design loaded to our main view
Code:
Bind this partial view with our model and receive the list pass from our controller using the code below.
• @model IEnumerable<UsingPartialView_MVC.Models.myModels>
And drop into C# code from the view and use for each loop to populate list to our table.
Full Code:
@model IEnumerable<UsingPartialView_MVC.Models.myModels>
<table class="table table-bordered">
<thead>
<tr>
<th>Name</th>
<th>Last</th>
<td>Number</td>
</tr>
</thead>
<tbody>
@foreach(var data in Model)
{
<tr>
<td>@data.Name</td>
<td>@data.LastName</td>
<td>@data.Number</td>
</tr>
}
</tbody>
</table>
From your HomeController create a list of sample data
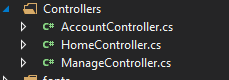
Code:
myModels[] model = new myModels[]
{
new myModels { Name="Regie",LastName="Baquero", Number="12345"},
new myModels { Name="Regie1",LastName="Baquero", Number="12346"},
new myModels { Name="Regie2",LastName="Baquero", Number="12347"},
new myModels { Name="Regie3",LastName="Baquero", Number="12348"},
new myModels { Name="Regie4",LastName="Baquero", Number="12349"}
};
myModels[] model1 = new myModels[]
{
new myModels { Name="Regie5",LastName="Baquero", Number="12310"},
new myModels { Name="Regie6",LastName="Baquero", Number="12311"},
new myModels { Name="Regie7",LastName="Baquero", Number="12312"}
};
Then create a method that will pass the sample data above to our partial view and return the partial view to our main view.
public PartialViewResult GetData1()
{
return PartialView("_patialview", model);
}
public PartialViewResult GetData2()
{
return PartialView("_patialview", model1);
}
In your index create your main view declare this script to enable Jquery
• <script src=”//ajax.googleapis.com/ajax/libs/jquery/1.9.1/jquery.min.js” type=”text/javascript”></script>
• <script src=”/Scripts/jquery.unobtrusive-ajax.js” type=”text/javascript”></script>
To install jquery.unobstrusive-ajax.js, I used the NuGet Package manager console. To open NuGet Console navigate to tools->NuGet Package Manager->Package Manager Console.
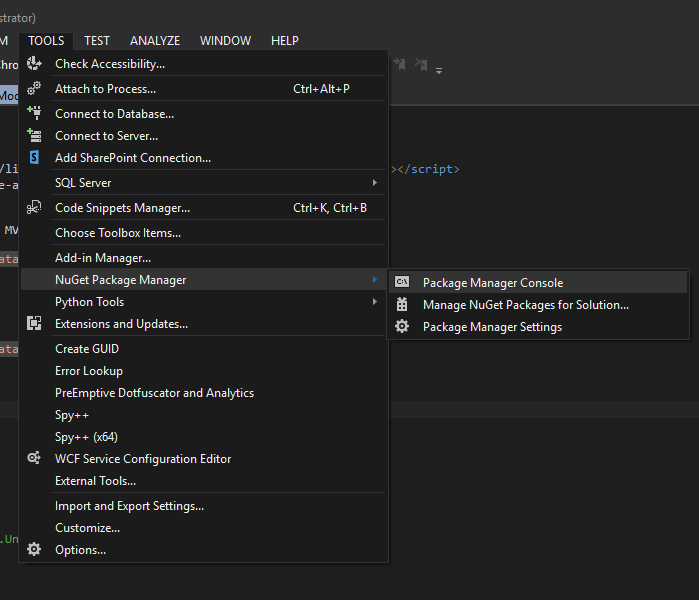
And then execute command: PM> Install-Package Microsoft.jQuery.Unobtrusive.Ajax
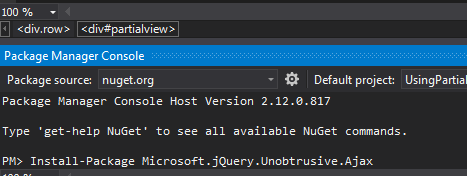
Create Ajax Helper
- HttpMethod: “GET”
- UpdateTargetId: DIV location where we want to put our partial view
- InsertionMode: Replace all element inside a DIV
@Ajax.ActionLink("Get Data1", "GetData1", new AjaxOptions()
{
HttpMethod = "GET",
UpdateTargetId = "partialview",
InsertionMode = InsertionMode.Replace
})
@Ajax.ActionLink("Get Data2", "GetData2", new AjaxOptions()
{
HttpMethod = "GET",
UpdateTargetId = "partialview",
InsertionMode = InsertionMode.Replace
})
Then we will create an empty DIV that will serve as the container for our partial view.
<div id="partialview">
</div>
Final Output:
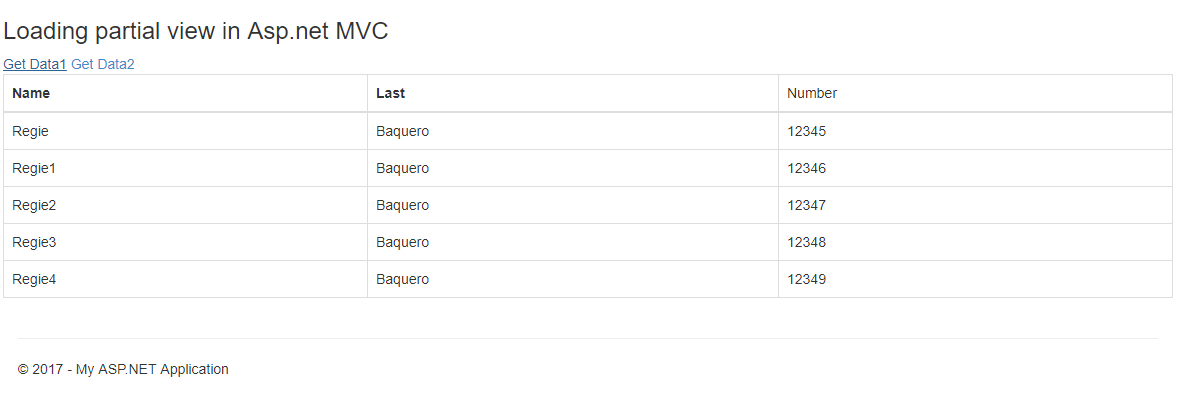
Code Summary
myModel:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace UsingPartialView_MVC.Models
{
public class myModels
{
public string Name { get; set; }
public string LastName { get; set; }
public string Number { get; set; }
}
}
_partialview:
@model IEnumerable<UsingPartialView_MVC.Models.myModels>
<table class="table table-bordered">
<thead>
<tr>
<th>Name</th>
<th>Last</th>
<td>Number</td>
</tr>
</thead>
<tbody>
@foreach(var data in Model)
{
<tr>
<td>@data.Name</td>
<td>@data.LastName</td>
<td>@data.Number</td>
</tr>
}
</tbody>
</table>
HomeController:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using UsingPartialView_MVC.Models;
namespace UsingPartialView_MVC.Controllers
{
public class HomeController : Controller
{
myModels[] model = new myModels[]
{
new myModels { Name="Regie",LastName="Baquero", Number="12345"},
new myModels { Name="Regie1",LastName="Baquero", Number="12346"},
new myModels { Name="Regie2",LastName="Baquero", Number="12347"},
new myModels { Name="Regie3",LastName="Baquero", Number="12348"},
new myModels { Name="Regie4",LastName="Baquero", Number="12349"}
};
myModels[] model1 = new myModels[]
{
new myModels { Name="Regie5",LastName="Baquero", Number="12310"},
new myModels { Name="Regie6",LastName="Baquero", Number="12311"},
new myModels { Name="Regie7",LastName="Baquero", Number="12312"}
};
public ActionResult Index()
{
return View();
}
public PartialViewResult GetData1()
{
return PartialView("_patialview", model);
}
public PartialViewResult GetData2()
{
return PartialView("_patialview", model1);
}
}
}
Index.chtml:
@{
ViewBag.Title = "Home Page";
}
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.9.1/jquery.min.js" type="text/javascript"></script>
<script src="/Scripts/jquery.unobtrusive-ajax.js" type="text/javascript"></script>
<div class="row">
<h3>Loading partial view in Asp.net MVC</h3>
@Ajax.ActionLink("Get Data1", "GetData1", new AjaxOptions()
{
HttpMethod = "GET",
UpdateTargetId = "partialview",
InsertionMode = InsertionMode.Replace
})
@Ajax.ActionLink("Get Data2", "GetData2", new AjaxOptions()
{
HttpMethod = "GET",
UpdateTargetId = "partialview",
InsertionMode = InsertionMode.Replace
})
<div id="partialview">
</div>
</div>
This is how we Create and Render Partial Views in ASP NET MVC. Now, you may test your web application by pressing F5 from your keyboard. Thank you for reading..
Enjoy coding!!!