The process of encapsulating one or more elements within a physical or logical container is characterized as encapsulation. Encapsulation prohibits access to implementation details in object-oriented programming.
C# also has a feature called abstraction, which is related to encapsulation. Abstraction is a technique for concealing undesired information. Encapsulation, on the other hand, is a way of hiding data in a single entity or unit as well as a method of protecting information from the outside.
Access specifiers are used to implementing encapsulation. The scope and visibility of a class member are defined by an access specifier. Below is the list of access specifiers cover in this tutorial.
- Public
- Private
- Internal
I. Public Access Specifier
The most common access specifier in C# is public. It can be accessed from any place, implying that accessibility is unrestricted. A public specifier is available both inside and outside the class. Any other code in the same assembly or another assembly that references it can access the type or member.
Note: I created a console application to run the example provided below.
Below is a sample implementation:
class Encapsulate
{
public string FirstName;
public string LastName;
public string concat()
{
return FirstName + " " + LastName;
}
public void Output()
{
Console.WriteLine("First Name: {0}", FirstName);
Console.WriteLine("Last Name: {0}", LastName);
Console.WriteLine("Full Name: {0}", concat());
}
}
class Program
{
static void Main(string[] args)
{
Encapsulate enc = new Encapsulate();
enc.FirstName = "FreeCode";
enc.LastName = "Spot";
enc.Output();
Console.ReadLine();
}
}
If you run this code, you will see this output.
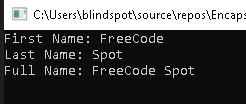
In the example above the variable from Encapsulate Class is declared as public, So they can be accessed from outside the class, which in this example it’s the Main() method. Even the Output() and the concat() method can access from the Main(), which is already outside the Encapsulate Class. This is how the class is called from my example above.
Encapsulate enc = new Encapsulate();
enc.FirstName = "FreeCode";
enc.LastName = "Spot";
enc.Output();
II. Private Access Specifier
A class’s member variables and member functions can be hidden from other functions and objects using the private access specifier. Only functions belonging to the same class have access to the class’s private members. Even a class instance is unable to access its private members.
If you change the variable FirstName and LastName from public specifier to private. You won’t be able to access it from outside its class. See the image below.
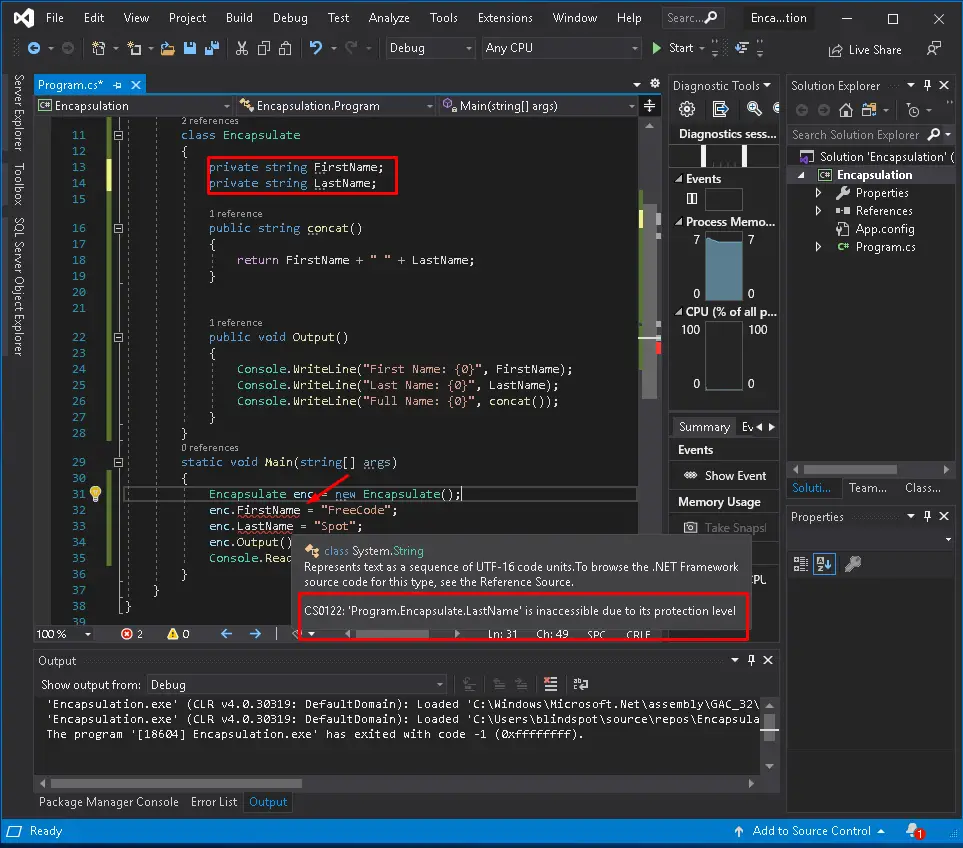
To create a workaround to use a private specifier, We need to create a public class that will collect the details from a user and assigns them to the First Name and Last Name variable from within the Encapsulate class. Instead of assigning value to the variable directly, we will call the public class that we will create. See the example below.
Here’s how you can implement a private specifier:
class Encapsulate
{
private string FirstName;
private string LastName;
public void Collect()
{
Console.WriteLine("First Name: ");
FirstName = Console.ReadLine();
Console.WriteLine("Last Name: ");
LastName = Console.ReadLine();
}
public string concat()
{
return FirstName + " " + LastName;
}
public void Output()
{
Console.WriteLine("First Name: {0}", FirstName);
Console.WriteLine("Last Name: {0}", LastName);
Console.WriteLine("Full Name: {0}", concat());
}
}
class Program
{
static void Main(string[] args)
{
Encapsulate enc = new Encapsulate();
//enc.FirstName = "FreeCode";
//enc.LastName = "Spot";
enc.Collect();
enc.Output();
Console.ReadLine();
}
}
Below is the image output for this implementation:
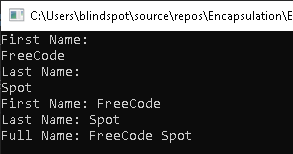
III. Internal Access Specifier
A class’s member variables and member functions can be exposed to other functions and objects in the current assembly using the internal access specifier. To put it another way, any member with an internal access specifier can be accessed from any class or method specified within the application in which it is defined.
Here’s how you can implement an internal specifier:
class Encapsulate
{
internal string FirstName;
internal string LastName;
public string concat()
{
return FirstName + " " + LastName;
}
public void Output()
{
Console.WriteLine("First Name: {0}", FirstName);
Console.WriteLine("Last Name: {0}", LastName);
Console.WriteLine("Full Name: {0}", concat());
}
}
class Program
{
static void Main(string[] args)
{
Encapsulate enc = new Encapsulate();
enc.FirstName = "FreeCode";
enc.LastName = "Spot";
enc.Output();
Console.ReadLine();
}
}
Below is the image output for this implementation:
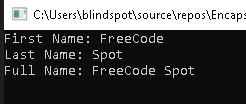
Summary
In this tutorial, we have discussed C# encapsulation and created a sample implementation of the common specifiers used in C#. Hopefully, this helps you understand more about when to use a public, private or internal specifier.
Visit Freecode Latest Blogs for more programming tutorials.
KEEP CODING!